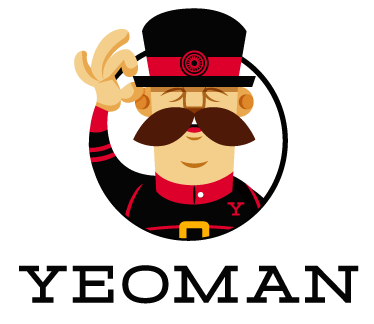
Easy app scaffolding with Yeoman
Ever wondered that the most time consuming tasks are the one you have done numerous times on numerous projects, and why the hell am i doing it again?
The most basic of examples of this is Scaffolding an app, you have to do a lot of things, repetitive things to get the app structure up to speed. This is an even bigger problem with NodeJS projects, and they do not have a sort of “Pre-defined / Fixed structure” if i may.
But that may be the thing of the past, because there’s a new sheriff in town, to get the burden off of all your trivial and important task related stuff, and do so with the ease and panache that will make you a believer.
Routing
, Dependency management
, packaging
, deployment
all taken care of by this nifty tool, which should be in every developers tool kit.
What is Yeoman?
Yeoman is an app scaffolding tool that helps you kickstart new projects, prescribing best practices and tools to help you stay productive. Yeoman provides a workflow to improve productivity and ease of development.
Yeoman itself comprises of three main components:-
Grunt/Gulp
: The build system to build/preview/test your appBower
: Frontend dependency management toolYeoman
: for scaffolding out apps, writing grunt tasks and bower dependencies
Enough talk! Show me the code
In order to use Yeoman you need to have NodeJS
installed, NodeJS
comes with the super awesome NPM (Node Package Manager)
which allows us to use many such tools as Yeoman, Grunt and Bower.
Assuming you have NodeJS Installed, install Yeoman as follows:
[js]
npm install -g yo
[/js]
The -g
denotes that this module will be installed globally and will be available from the command-line. Sweet.
Now, Yeoman is a very versatile tool, i.e. its functionality can be extended by anyone, this is possible because of the integration of something called Generators
which allow yeoman to scaffold apps quickly.
There are a lot of generators available, for multiple languages and frameworks, Frontend, backend etc.
Since we already have node installed, why not scaffold a NodeJS
app complete with full AngularJS
integration.
Generators are the fuel of Yeoman, to build our app we’ll use a generator called generator-angular-fullstack
complete with MongoDB
, Mongoose
, SocketIO
, Passport
integration out of the box 😉
Let’s install it now:
[js]
npm install -g generator-angular-fullstack
[/js]
This will install your generator to be used by yeoman. Next we need to create a project directory in which Yeoman will scaffold our app.
[js]
mkdir my-yeoman-project
cd my-yeoman-project
[/js]
Let’s generate our app by running the angular-fullstack
with yo
:
[js]
yo angular-fullstack
[/js]
This will launch you into a interactive app configuration setup, where you can easily add/remove options upon your own requirements.
It will ask you certain configuration for both client and server:
Client
# Scripts
– Javascript / Coffeescript
# Markup
– HTML / Jade
# Stylesheets
– CSS / Sass / Less
# Angular router
– ngRoute / uiRouter
Server
# Use MongoDB with Mongoose
– (Requires mongodb installed and running)
# Basic Authentication
– Add basic Login / Register schemes
# Additional OAuth Strategies using Passport
– Google / Facebook / Twitter
# SocketIO
All this will give us a directory structure like following:
[js]
_
├─ client
│ ├─ app – App specific components
│ ├─ assets – Custom assets: fonts, images, etc…
│ ├─ components – Our reusable components
│
├─ e2e – Protractor end to end tests
│
└─ server
├─ api – Our apps server api
├─ auth – For handling authentication
├─ components – Our reusable or app-wide components
├─ config – Where we do the bulk of our apps configuration
│ └─ local.env.js – Environment variables
│ └─ environment – Configuration specific to the node environment
└─ views – Server rendered views
[/js]
With all the required Grunt tasks to combine
, minify
, building
etc. and cleanly written angular components
and services
to help us quickly get started.
Even more ..
The above app structure is really good because we only have a limited number of files within an Entity
, there can be multiple entities with separate controllers, models etc.
Now how do i add more entities, surely copying and pasting the files would be one way to do it, but folks at yeoman have this covered as well.
The backend entities are called endpoints, which include the controllers
, models
(with full CRUD impemented), and RESTful
routing. To add more endpoints we simply have to tell yeoman
to generate it for us, like so:
[js]
yo angular-fullstack:endpoint your-endpoint-name
[?] What will the url of your endpoint to be? (/api/your-endpoint-name)
create server/api/your-endpoint-name/index.js
create server/api/your-endpoint-name/your-endpoint-name.controller.js
create server/api/your-endpoint-name/your-endpoint-name.model.js
create server/api/your-endpoint-name/your-endpoint-name.socket.js
create server/api/your-endpoint-name/your-endpoint-name.spec.js
[/js]
This will create a number of files for us to use directly including tests
and SocketIO
file (if configured).
In fact there are many commands for us to do a number of tasks, both for generating code templates for Backend as well as the frontend.
Angular Routes
[js]
yo angular-fullstack:route myroute
[?] Where would you like to create this route? client/app/
[?] What will the url of your route be? /myroute
create client/app/myroute/myroute.js
create client/app/myroute/myroute.controller.js
create client/app/myroute/myroute.controller.spec.js
create client/app/myroute/myroute.html
create client/app/myroute/myroute.scss
[/js]
Angular Controller
[js]
yo angular-fullstack:controller user
[?] Where would you like to create this controller? client/app/
create client/app/user/user.controller.js
create client/app/user/user.controller.spec.js
[/js]
Directives / filters
[js]
yo angular-fullstack:directive myDirective
[?] Where would you like to create this directive? client/app/
[?] Does this directive need an external html file? Yes
create client/app/myDirective/myDirective.directive.js
create client/app/myDirective/myDirective.directive.spec.js
create client/app/myDirective/myDirective.html
create client/app/myDirective/myDirective.scss
[/js]
[js]
yo angular-fullstack:filter myFilter
[?] Where would you like to create this filter? client/app/
create client/app/myDirective/myFilter.filter.js
create client/app/myDirective/myFilter.filter.spec.js
[/js]
Similar commands for Services
, decorators
etc.
Running / Testing
To run our app we dont need to do anything special, Grunt will figure this out ..
we simply have to do:
[js]
//DEVELOPMENT
grunt serve
//DISTRIBUTION/PRODUCTION ENV
grunt serve:dist
[/js]
This will run our app with desired environment and even open up a browser for us 😉 cool huh!
Running tests
To run all our tests run:
[js]
//RUN ALL THE TESTS
grunt test
//ONLY SERVER TESTS
grunt test:server
//ONLY CLIENT TESTS
grunt test:client
[/js]
Simple 🙂
Deployment
All the code written means nothing if it’s not deployed. Yeoman also provides us extremely simple deployment options.
Yeoman currently provides easy deployment for OpenShift
and Heroku
. To deploy to either of these services just do:
[js]
//HEROKU
yo angular-fullstack:heroku
//OPENSHIFT
yo angular-fullstack:openshift
[/js]
(Assuming all the configuration is already done once)
All the power Yeoman
provides us is extremely overwhelming at first, but once you get in the groove, it is a brilliant and nifty little tool in our development workflow which help us save time and energy and allows us to focus on important tasks and problems.
Learn more about Yeoman and angular-fullstack.
Till next time,
Ciao