Demystifying Freezed Annotations: Mastering Data Modeling for Complex State in Flutter 🥶
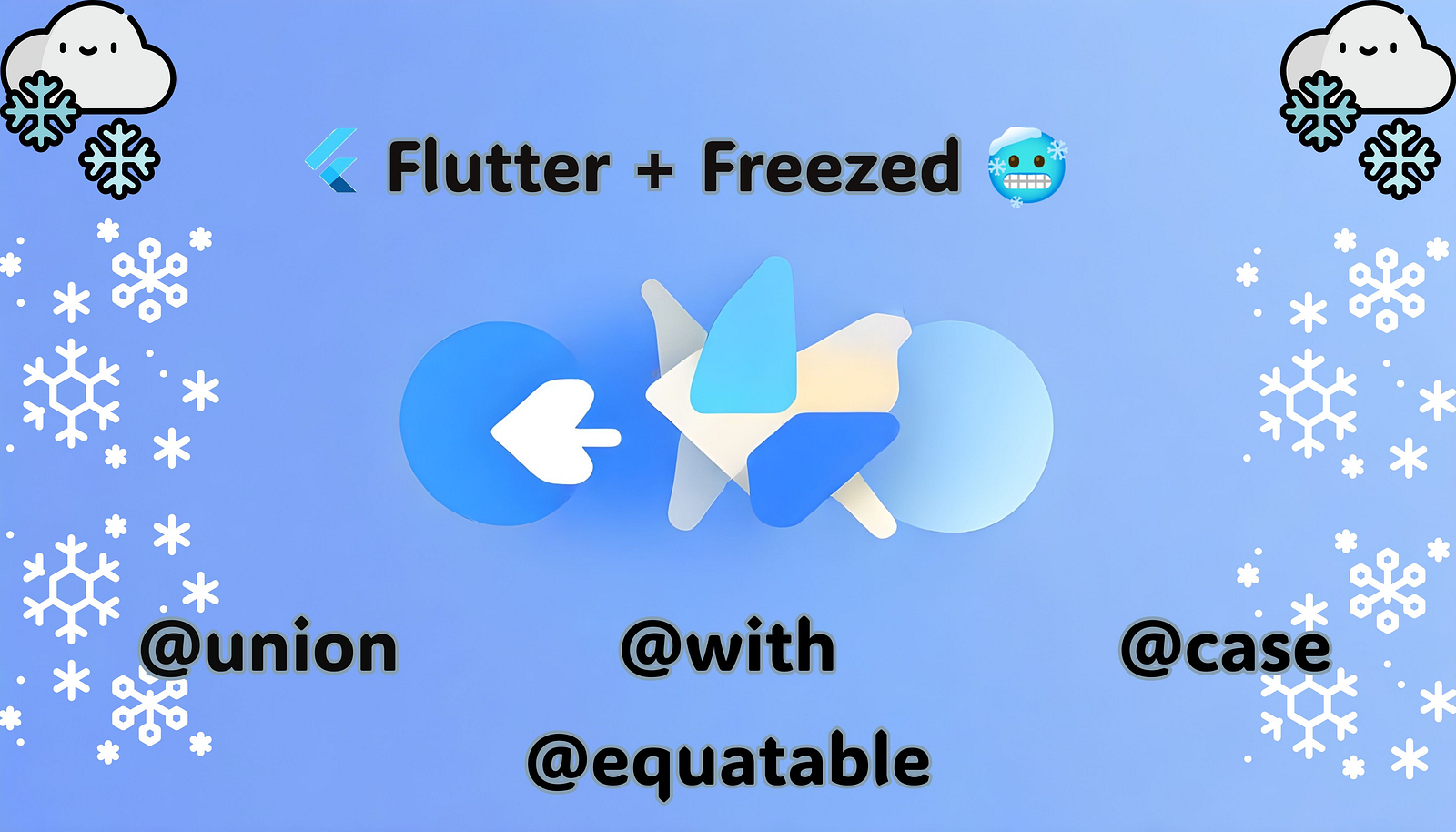
The world of Flutter development thrives on well-structured and maintainable code. When it comes to data modeling for intricate state management, the freezed
package emerges as a champion. It offers a powerful annotation toolkit that empowers you to create robust and expressive data classes. This blog post delves into four key annotations – @Union
, @with
, @case
, and @equatable
– and explores how they work together to elevate your state management game in Flutter.
The Power of Immutability: Why Freezed?
Traditional Dart classes are mutable, meaning their internal data can be altered after creation. This mutability can introduce inconsistencies and unforeseen behavior in your application, leading to debugging challenges. Freezed tackles this by promoting immutability. When you use freezed
it to define a data class, it automatically generates an immutable counterpart with a fixed set of properties. This ensures data integrity and simplifies reasoning about your application’s state throughout its lifecycle.
Introducing the Key Players: Freezed Annotations
Freezed provides a comprehensive set of annotations that work in concert to streamline your data modeling process, particularly for complex state scenarios. Let’s explore four crucial ones:
- @Union: Define Sealed Union Types
This annotation allows you to create a set of possible states for your data class, representing different scenarios within your application. Imagine aUserState
union type with states likeUserInitial
,UserLoading
,UserLoaded
, andUserError
. Each state holds relevant information specific to its purpose.
// This code demonstrates the use of @Union to define sealed union types
@freezed
abstract class UserState with _$UserState {
const factory UserState.initial() // Represents the initial state of the user
= UserInitial;
const factory UserState.loading() // Represents a loading state while fetching user data
= UserLoading;
const factory UserState.loaded(User user) // Represents a state where user data is loaded successfully
= UserLoaded;
const factory UserState.error(String message) // Represents an error state with an error message
= UserError;
}
- @with: Combine Existing Classes for Enhanced Data
This annotation allows you to combine properties from multiple existing data classes into a new class. This is particularly useful when you need to extend functionality or create a data class with properties from various sources.
// This code demonstrates the use of @with to combine properties from existing classes
@freezed
abstract class ProductDetails with _$ProductDetails {
const factory ProductDetails.fromProduct(Product product)
@With<Product>() // Combine properties from the Product class
=> ProductDetails(
id: product.id,
name: product.name,
description: product.description,
// Add additional properties specific to product details
);
const factory ProductDetails({
required String id,
required String name,
required String description,
// Additional details specific to product details
}) = _ProductDetails;
}
- @case: Customize Generated Constructor Names
By default,freezed
generates constructor names based on the class name and state names. However,@case
allows you to customize these names for improved readability and clarity in your code.
// This code demonstrates the use of @case to customize constructor names
@freezed
abstract class CartEvent with _$CartEvent {
@case('add_to_cart') // Customize constructor name for better readability
const factory CartEvent.addItem(Product product) = AddItem;
@case('remove_from_cart') // Customize constructor name for better readability
const factory CartEvent.removeItem(Product product) = RemoveItem;
}
- @Equatable (from the
equatable
package): Ensure Proper Object Comparison
Immutability is valuable, but effectively comparing data objects for equality is also crucial for state management logic.@Equatable
(used alongsidefreezed
) assists in generating methods like==
andhashCode
based on the data class properties, ensuring proper object comparison during state updates.
// This code demonstrates the use of @Equatable for proper object comparison
@freezed
@Equatable
abstract class Filter with _$Filter {
const factory Filter({
@Default(VisibilityFilter.all) VisibilityFilter visibilityFilter,
}) = _Filter;
@override
List<Object?> get props => [visibilityFilter]; // Define properties used for equality comparison
}
enum VisibilityFilter { all, active, completed }
Benefits of Using These Annotations with Freezed
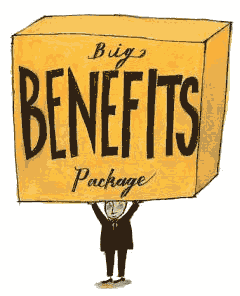
- Expressive Data Modeling: Define clear and concise states for your application, making your state management logic more readable and maintainable.
- Reduced Boilerplate: These annotations eliminate the need for manually writing constructors, copy methods, and equality checks in many cases.
- Improved Readability: Customize constructor names and leverage clear state definitions to enhance code clarity for both you and your team.
- Enhanced Type Safety: By defining data structures with annotations, you benefit from Dart’s static type checking, helping to prevent runtime errors.
- Stronger Testability: Immutable data classes and proper object comparison using
@Equatable
lead to significantly more reliable and testable code.
Conclusion: Building a Solid Foundation for State Management
By leveraging annotations like @Union
, @with
, @case
, and @equatable
in conjunction with freezed
, you can construct well-structured and expressive data classes that form the foundation for robust and maintainable state management in your Flutter applications. With cleaner code, improved readability, enhanced type safety, and stronger testability, freezed
you can focus on crafting exceptional user experiences while keeping your state logic clear and predictable. So, embrace the power of annotations and take your Flutter development to the next level!