Custom Exceptional Handling using ControllerAdvice
Here, we can see in the above image that the product is not present for this product ID. But we are getting proper 200 status. which is not a proper understandable response.
Let’s understand why we need Custom Exceptional Handing
Using @ControllerAdvice
in Spring allows you to create a centralized exception handler that can handle exceptions thrown by controllers in your application. This is a powerful feature for custom exception handling. Here’s a step-by-step guide on how to implement custom exception handling using @ControllerAdvice
in Spring:
Step 1: Create Custom Exception Classes
Create custom exception classes that extend RuntimeException
Or a suitable base exception class. For example:
public class ProductNotFoundException extends RuntimeException {
public ProductNotFoundException(String message) {
super(message);
}
}
public class InvalidRequestException extends RuntimeException {
public InvalidRequestException(String message) {
super(message);
}
}
You can create as many custom exception classes as needed for different error scenarios.
Step 2: Create a Global Exception Handler
Create a global exception handler class annotated with @ControllerAdvice
. This class will contain methods to handle various exceptions.
Here’s an example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ProductNotFoundException.class)
public ResponseEntity<String> handleProductNotFoundException(ProductNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
@ExceptionHandler(InvalidRequestException.class)
public ResponseEntity<String> handleInvalidRequestException(InvalidRequestException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.BAD_REQUEST);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleOtherExceptions(Exception ex) {
return new ResponseEntity<>("An error occurred: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
In this example, we’ve created three exception-handling methods: one for handling ProductNotFoundException
, one for handling InvalidRequestException
, and another for handling general exceptions. These methods return appropriate HTTP response statuses and error messages.
Step 3: Throw Custom Exceptions in Your Controllers
In your controller methods, throw custom exceptions when needed. For example:
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/{id}")
public ResponseEntity<Product> getProductById(@PathVariable Long id) {
Product product = productService.getProductById(id);
if (product == null) {
throw new ProductNotFoundException("Product not found with ID: " + id);
}
return ResponseEntity.ok(product);
}
@PostMapping
public ResponseEntity<String> createProduct(@RequestBody Product product) {
if (product == null) {
throw new InvalidRequestException("Invalid product request");
}
productService.createProduct(product);
return ResponseEntity.status(HttpStatus.CREATED).body("Product created successfully");
}
// Other controller methods
}
In this example, when a product is not found (getProductById
) or an invalid request is made (createProduct
), we throw custom exceptions (ProductNotFoundException
and InvalidRequestException
).
Step 4: Test the Exception Handling
Now, when you access your RESTful endpoints and an exception is thrown, the global exception handler will catch the exception and return the appropriate HTTP status and error message.
For example, if you make a GET request to /api/products/123
, and a product with ID 123 is not found, the response will have a status code of 404 (NOT FOUND) and an error message like “Product not found with ID: 123.”
By following these steps, you’ve added custom exception handling using @ControllerAdvice
in your Spring Boot application, allowing you to handle specific exceptions gracefully and provide meaningful error responses to clients.
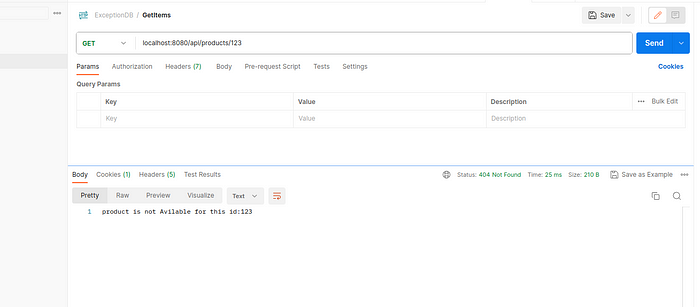
After we fix this issue, we’ll be getting a proper response. You can have a look at the code here on github.
You can comment down below or email me your queries.