Introduction to Gulp.js: Beginner’s Guide
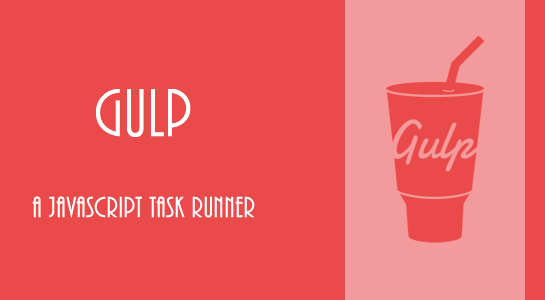
GULP FOR BEGINNERS
INTRODUCTION
Gulp is an open-source Javascript toolkit and task runner which is built on Node.js and NPM and used for the optimization of time-consuming and repetitive tasks. Hundreds of plugins are available for different tasks You don’t have to program everything by yourself, there are nearly 800 plugins ready for Gulp.js. But even more, Node.js modules can be used to build the perfect build and development process for your needs.
WHY GULP JS?
As a Developer or Web Designer, you will likely need plenty of things to build a modern website: a development server, a preprocessor for your Sass, Less, or Stylus files, automation to bundle your JavaScript, tools to optimize your code, to compress, compile or move things around. And if you change something, you want your files to update automatically, or refresh the browser. You don’t want to do this manually
Installation of Gulp
STEP-1: First we need node js which you can download here. Follow the steps and install Nodejs and in the command prompt or terminal enter the following command
Node -v
it will show you the version
STEP2:Now after the Installation of Nodejs. Install Gulp js globally in the same way. Enter the following command in the command prompt or terminal
npm install gulp-cli -g
In the same way, check it by entering
gulp -v
It will show the version.
Setting Up Your Project
Before setting up the project let’s learn a few things more
Build System
A Build System is referred to as a collection of tasks (collectively called task runners), which automate repetitive work.
gulpfile.js
A gulpfile is a file in your project directory titled gulpfile.js that automatically loads when you run the gulp command.
src (source) & dist (distribution)
The src folder will contain all your source files such as plain JavaScript, uncompressed (s) CSS, and many more. The dist folder will be completely built up by Gulp. You can always check if gulp is working fine by deleting the dist folder and rebuilding it gulp, which you’re going to learn.
Installation for Project
To set up your project on your computer, create a folder called “Webpage” for example. You can also create a theme folder if you’re using CMS like Drupal. Then initialize a node project by running the usual command. Create a package.json file in your project directory by
npm init
Install the gulp package in your devDependencies
npm install --save-dev gulp
Verify your gulp version
gulp --version
Create a gulpfile
Create a file gulpfile.js and add the following code for testing
function defaultTask(cb) {
// place code for your default task here
cb();
}
exports.default = defaultTask
Run the gulp command in your project directory:
gulp
The default task will run and do nothing. That means gulp is perfectly working. Now we can go further. If not, Follow the same steps again The Webpage folder contains the following sub-folders and files –
- index.html — your main HTML file
- package.json — contains all the npm packages to install when you run npm install.
- gulpfile.js — contains the config and runs all the Gulp tasks
- /src — working folder, you will edit SCSS/JS files in here
- /dist — Gulp will output files here, don’t edit these files
In your workflow, you will edit the HTML, SCSS, and JS files. Gulp will then detect any changes and compile everything. Then you will load your final CSS/JS files from the /dist folder in your index.html file.
Setting up Gulp for CSS & JS
Folder Structure
Note: Don’t create any files in the dist folder. It will be created automatically by the gulp task runner
Here, We are using gulp for CSS & JS optimization and will compress it via gulp. To use gulp-sass, you must install both gulp-sass itself and a Sass compiler. Enter the following command into your project terminal
npm install sass gulp-sass --save-dev
Import it into your Gulpfile.js
This is the SCSS code to be compiled into CSS.
const gulp = require('gulp'); const sass = require('gulp-sass')(require('sass')) gulp.task('styles', () => { return gulp.src('./src/sass/*.scss') .pipe(sass().on('error', sass.logError)) .pipe(gulp.dest('./dist/css/')); }); gulp.task('default', gulp.series(['styles']));
This is the gulpfile.js that contains our Gulp tasks
- gulp.task(‘default’, gulp.series([‘styles’]));
- The default task is a task that is executed if no task name is specified with Gulp CLI. It runs the clean and styles tasks in sequential order.
Gulp Watch Task (Important)
Now let’s begin to understand how we can use gulp.watch into our project. The Watch method is used to monitor your source files. When any changes to the source file are made, the watch will run an appropriate task. You can use the ‘default’ task to watch for changes to HTML, CSS, and JavaScript files. We can use gulp.watch() to automatically watch for changes. We can now use gulp.watch instead of gulp.task exports
Plugins for optimization of CSS & JS
- gulp-concat-css: This plugin is used to concatenate 2 or more CSS files into a single output file.
- gulp-cssnano: It is used for compressing CSS.
- gulp-minify: Js minify plugin for gulp.
npm install --save-dev gulp-concat-css
npm install gulp-cssnano --save-dev
npm install --save-dev gulp-minify
Define and import your gulpfile.js
const gulp = require('gulp');
const concatCss = require('gulp-concat-css');
const cssNano = require('gulp-cssnano')
const minify = require('gulp-minify');
const sass = require('gulp-sass')(require('sass'));
Our package.json file should look like this.
“devDependencies”: {
“gulp”: “^4.0.2”,
“gulp-concat-css”: “^3.1.0”,
“gulp-cssnano”: “^2.1.3”,
“gulp-minify”: “^3.1.0”,
“gulp-sass”: “^5.1.0”,
“sass”: “^1.57.1”
}
Use the below code to read and concat all the CSS files and JS files.
gulp.task('sass', function sassFunc(){ return gulp.src('./src/sass/*.scss') .pipe(sass()) .pipe(concatCss('custom.css')) .pipe(gulp.dest('./dist/css')) }); gulp.task('compress', function compressFunc() { return gulp.src('./dist/css/custom.css') .pipe(cssNano()) .pipe(gulp.dest('./dist/css')); }); gulp.task('compressJs', function() { gulp.src(['./src/js/*.js']) .pipe(minify()) .pipe(gulp.dest('./dist/js')) });
Gulp task is an asynchronous JavaScript function – a function that accepts an error-first callback or returns a stream, promise, event emitter, child process, or observable We have defined session-start and build-css tasks in the gulpfile.js. You can use any meaningful names for these tasks.
Gulp Watch
Define gulp.watch into your gulpfile.js
gulp.task('watch', function watchFunc() {
gulp.watch('./src/sass/*.scss', gulp.series('sass', 'compress'));
gulp.watch('./src/js/*.js', gulp.series('compressJs'));
});
There are two types of functions. Here we are using series function
- parallel(): Combines task functions and/or composed operations into larger operations that will be executed simultaneously.
- series(): Combines task functions and/or composed operations into larger operations that will be executed one after another, in sequential order.
Now run the following command into your terminal
gulp watch
You’ll see something like this. And After this, you can do your work while the gulp-watch will take care of converting and compressing your files. Don’t forget to off-gulp-watch after you finish your task.
Advantages
- Easy to code and understand
- The huge speed advantage over any other task runner
- There are a lot of plugins available which are simple to use and they are designed to do one thing at a time.
- Performs repetitive tasks repeatedly such as minifying stylesheets, compressing images, etc.
- If you’re using SASS, you don’t have to import files. You can directly create an scss file which will import into CSS by gulp.
Final Words
Gulp can be used to automatize a great number of menial tasks that are common during development. You should be able to use the final gulpfile.js as a basis for your project, with some slight modification, and you can find a plug-in for your particular need.
Gulp provides some useful plugins to work with HTML & CSS, JavaScript, and many more. A few examples are shown below
- autoprefixerIt automatically includes prefixes to CSS properties.
- gulp-responsiveIt generates responsive images for different devices
- gulp-uglifyMinify files with UglifyJS.
- gulp-imageminMinify PNG, JPEG, GIF, and SVG images
For more plugins. You can always visit the gulp plugin site