Event optimization using debounce
What is Debounce?
Debounce is an optimization technique to reduce the frequency of repetitive function calls.
It is especially suitable for cases where we have some function that can be called multiple times within a short duration, but only the latest execution result is required.
There are many ways to implement it. The general concept is we take a function and attach a timer to it. Now, whenever the method gets called, the timer starts, and the code executes when the timer finishes. If it gets called again before the ongoing timer completes, the previous call is rejected (the logic will not run), and the timer starts again, repeating the process. It happens till the timer completes. Then, only the logic is executed.
Let’s take an example to get more clarity.
Assume there is a search box in the UI. We want to make the search results look real-time. The code is such that for every input from the user, an API call is made to the server, and upon receiving a response, we show the result in the UI.
It is a perfect scenario for debounce as we want the search to appear in real-time, but only the result of the latest input is required. In short, we can skip the in-between API calls. Let’s see how.
I have created a simple app using React. Just like our example, it has a search input.
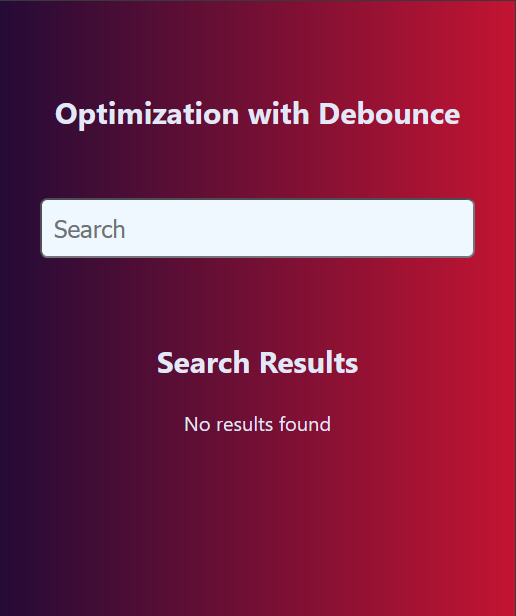
We also have a search component.
It has an input field, and on any change in the input, it calls the fetchData function. fetchData calls the API and sets the result to show in the UI.
The API that I’m using is for movies. So let’s search “Dark Knight”…
We have the results, but notice the number of API calls made. There are eleven API calls (one for each character).
Let’s use debounce here.
I have created a higher-order function (debounce). A higher-order function is a function that takes another function as an argument or returns a function.
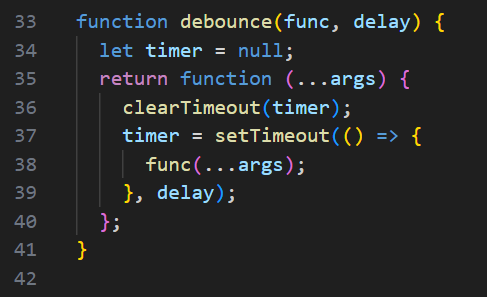
The debounce function takes two arguments –
- func (The function to debounce. We’ll call it the original function)
- delay (time for setTimeout)
Let’s go back to our Search component and make the necessary changes.
- We’ll create a debounced version of the fetchData function (debouncedFetchData).
- Whenever the input changes, call debouncedFetchData instead of fetchData.
Okay, now let’s try the search again.
This time, there were only four API calls compared to the eleven before.
Conclusion
Event handling is an integral part of front-end development. Anytime we work with repetitive events that we can group, debounce might fit the use case perfectly. It is simple to implement yet very effective, and it can save server costs and make the application more performant.
Please refer to our blogs for more insightful content.